Table of Contents
Hatched Rectangle
(defun hatched-rect3p-x (o s z spacing) (rect3p o s z) (let* ((n (floor (/ (x s) spacing))) (spacing (/ (x s) n))) (loop for x from 1 below n do (line3p (vec2 (+ (x o) (* x spacing)) (y o)) (vec2 (+ (x o) (* x spacing)) (+ (y o) (y s))) z)))) (defun hatched-rect3p-y (o s z spacing) (rect3p o s z) (let* ((n (floor (/ (y s) spacing))) (spacing (/ (y s) n))) (loop for y from 1 below n do (line3p (vec2 (x o) (+ (y o) (* y spacing))) (vec2 (+ (x o) (x s)) (+ (y o) (* y spacing))) z))))
Square
(with-scene (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.0 1.0) 0 0.1) (plot-pseudo3d "images/hatching_test/square.png"))
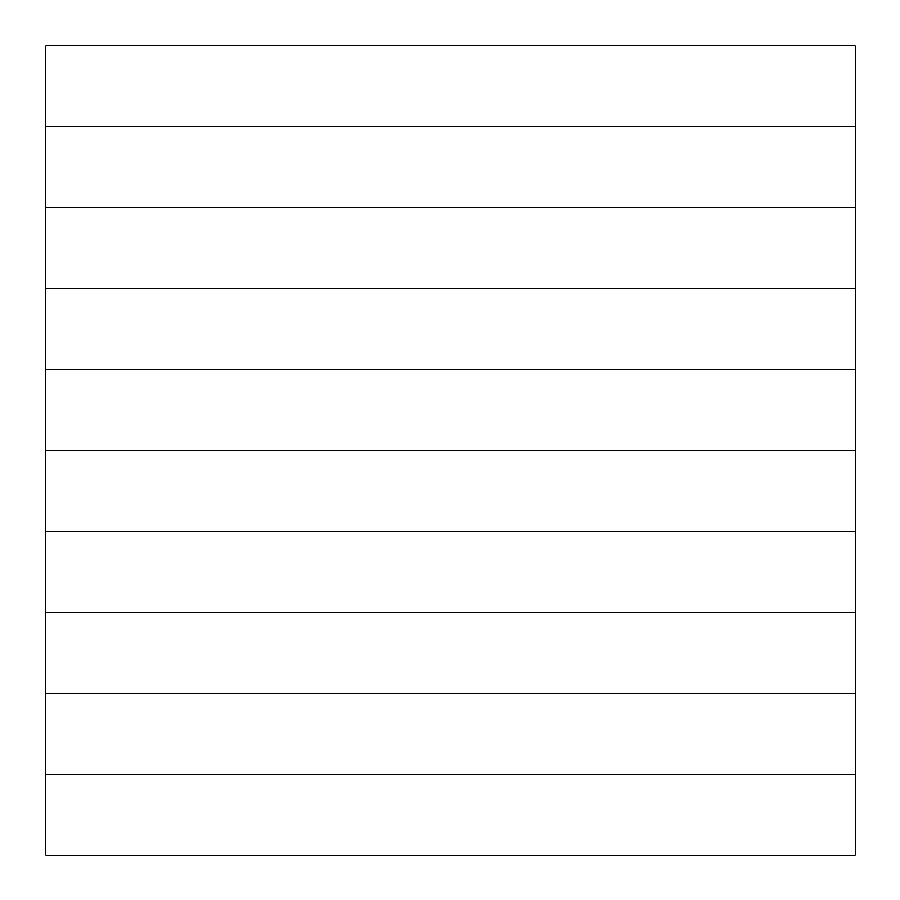
Repetition
(with-scene (translate-repeat 5 (vec2 2.0 0.0 0.0) (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.0 10.0) 0 0.1)) (plot-pseudo3d "images/hatching_test/repetition.png"))
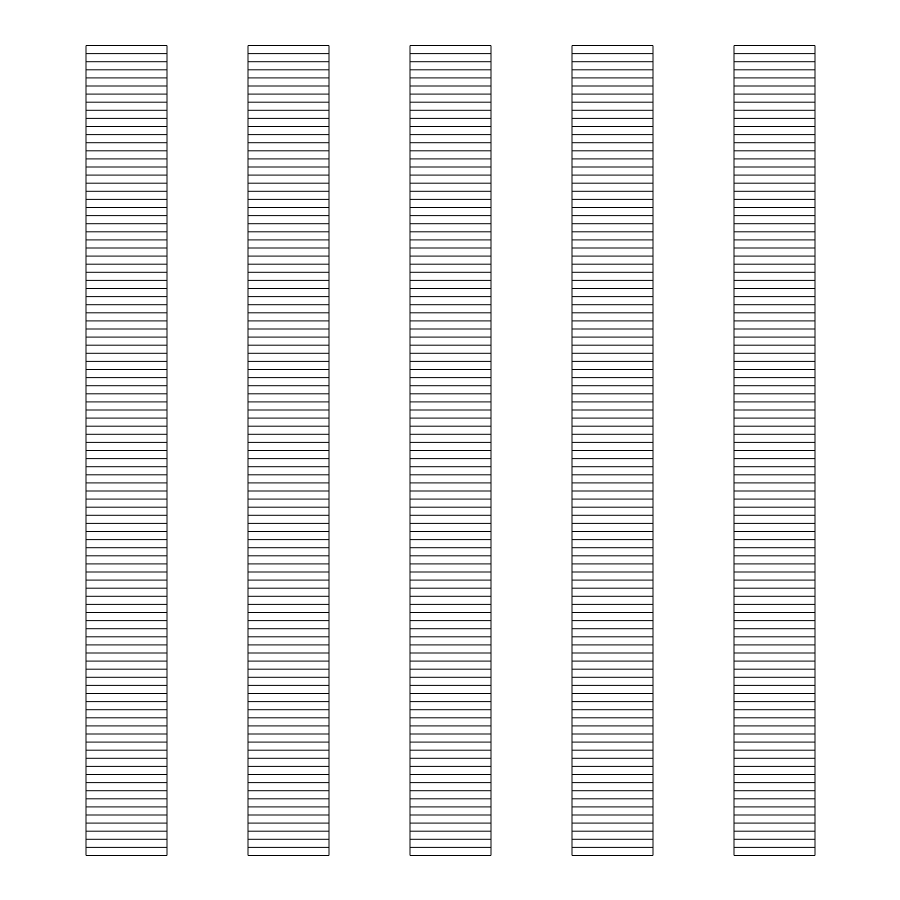
Combination
(with-scene (repeat 5 (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.0 10.0) 0 0.2) (move 1.0 0.0) (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.0 10.0) 0 0.1) (move 1.0 0.0)) (plot-pseudo3d "images/hatching_test/combination.png"))
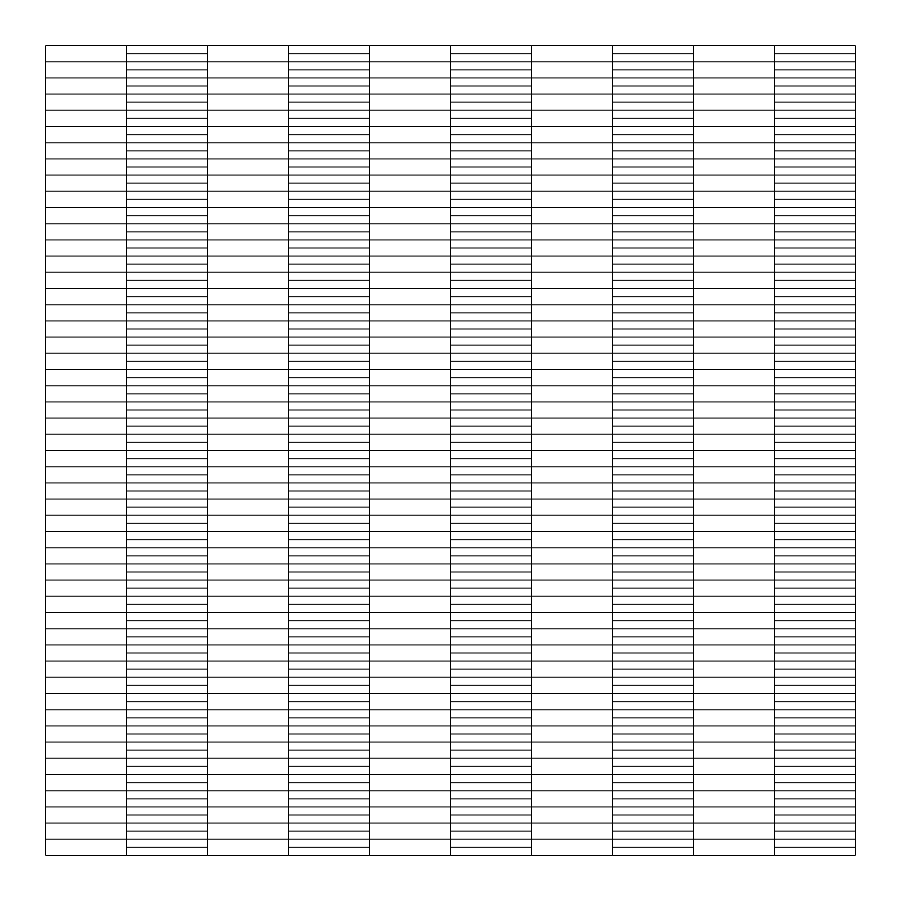
Gradient
(with-scene (dotimes (i 10) (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.0 10.0) 0 (/ 1.0 (1+ i))) (move 1.0 0.0)) (plot-pseudo3d "images/hatching_test/gradient.png"))
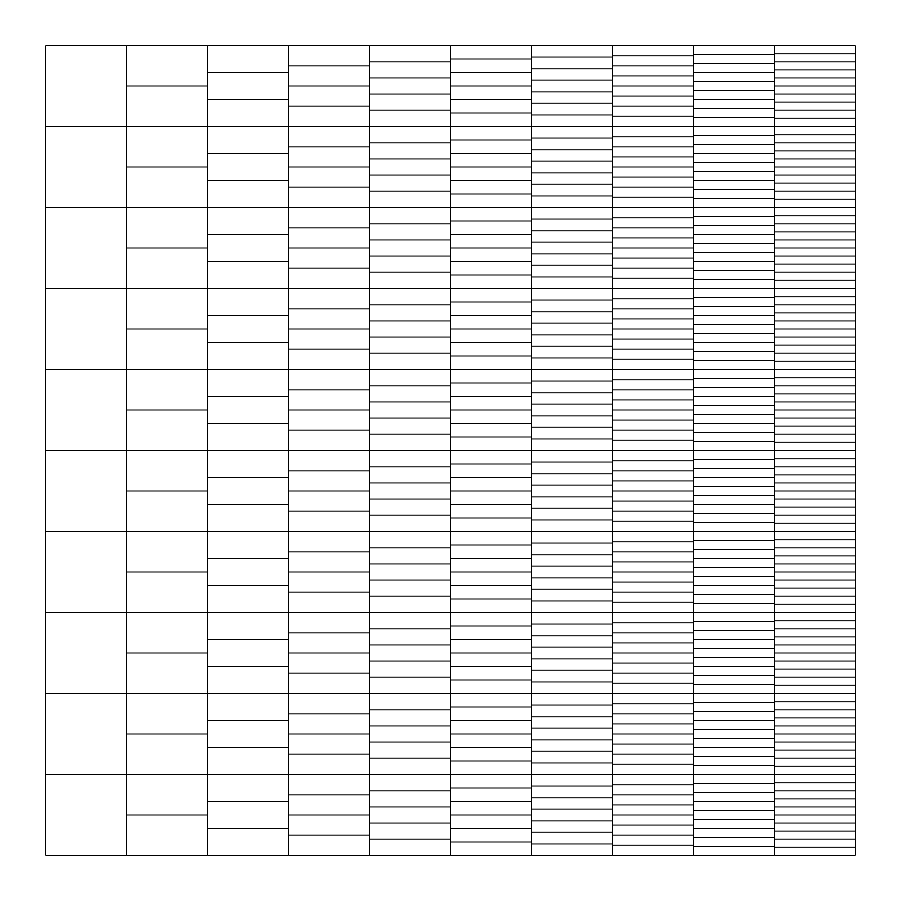
Overlay
(with-scene (loop for x from 0 below 8 do (hatched-rect3p-y (vec2 (* 2 x) 0.0) (vec2 1.0 16.0) 0 0.1)) (loop for x from 0 below 8 do (hatched-rect3p-y (vec2 0.0 (* 2 x)) (vec2 16.0 1.0) 1 0.2)) (loop for x from 0 below 4 do (hatched-rect3p-y (vec2 0.0 (* 4 x)) (vec2 16.0 1.0) -1 0.6)) (loop for x from 0 below 4 do (hatched-rect3p-y (vec2 (* 4 x) 0.0) (vec2 1.0 16.0) -2 0.05)) (plot-pseudo3d "images/hatching_test/overlay.png"))
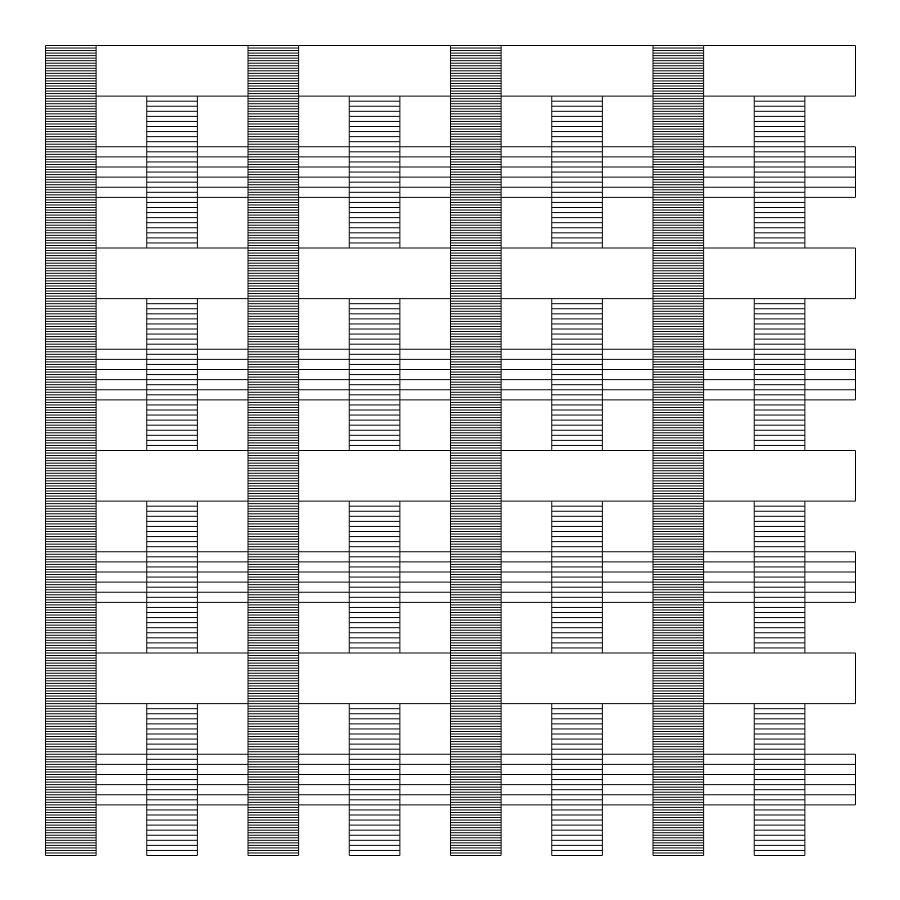
Random
(dotimes (i 5) (with-scene (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-y (vec2 x 0.0) (vec2 1.0 16.0) (rand% 0 1000) 0.1))) (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-y (vec2 0.0 x) (vec2 16.0 1.0) (rand% 0 1000) 0.2))) (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-y (vec2 0.0 x) (vec2 16.0 1.0) (rand% 0 1000) 0.4))) (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-y (vec2 x 0.0) (vec2 1.0 16.0) (rand% 0 1000) 0.05))) (plot-pseudo3d (format nil "images/hatching_test/random~d.png" i))))
Random, Two Axes
(dotimes (i 5) (with-scene (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-y (vec2 x 0.0) (vec2 1.0 16.0) (rand% 0 1000) 0.1))) (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-x (vec2 0.0 x) (vec2 16.0 1.0) (rand% 0 1000) 0.2))) (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-y (vec2 0.0 x) (vec2 16.0 1.0) (rand% 0 1000) 0.4))) (loop for x from 0 below 16 do (with-prob 0.5 (hatched-rect3p-x (vec2 x 0.0) (vec2 1.0 16.0) (rand% 0 1000) 0.05))) (plot-pseudo3d (format nil "images/hatching_test/random2_~d.png" i))))
Probabilistic Hatching
(defun phatched-rect3p-x (o s z spacing prob) (rect3p o s z) (let* ((n (floor (/ (x s) spacing))) (spacing (/ (x s) n))) (loop for x from 1 below n do (with-prob prob (line3p (vec2 (+ (x o) (* x spacing)) (y o)) (vec2 (+ (x o) (* x spacing)) (+ (y o) (y s))) z))))) (defun phatched-rect3p-y (o s z spacing prob) (rect3p o s z) (let* ((n (floor (/ (y s) spacing))) (spacing (/ (y s) n))) (loop for y from 1 below n do (with-prob prob (line3p (vec2 (x o) (+ (y o) (* y spacing))) (vec2 (+ (x o) (x s)) (+ (y o) (* y spacing))) z)))))
(with-scene (loop for x from 0 below 16 do (phatched-rect3p-y (vec2 (* x 0.5) 0.0) (vec2 0.5 8.0) 0 0.1 (sqrt (/ 1.0 (1+ x))))) (plot-pseudo3d "images/hatching_test/gradient2.png"))
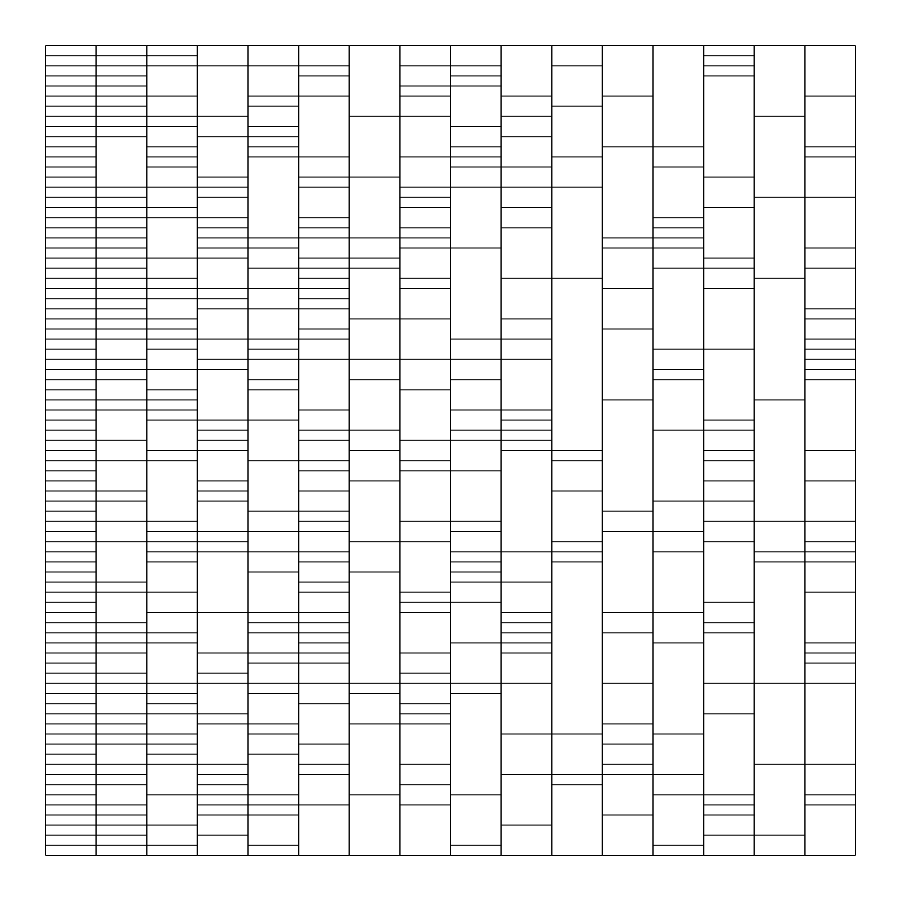
Grids
(with-scene (translate-repeat 5 (vec2 0.0 2.0) (translate-repeat 5 (vec2 2.0 0.0) (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.0 1.0) 0 0.1))) (plot-pseudo3d "images/hatching_test/repetition2.png"))
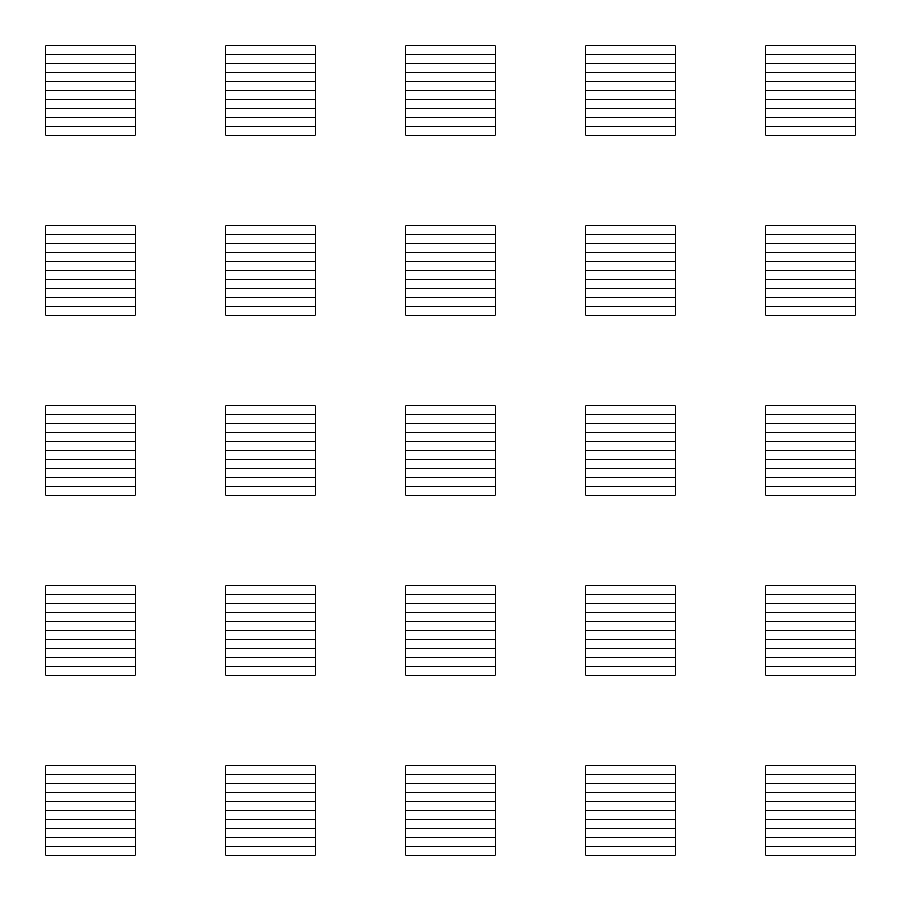
(defmacro translate-grid (n offset &rest body) `(translate-repeat ,n (vec2 0.0 ,offset) (translate-repeat ,n (vec2 ,offset 0.0) ,@body))) (with-scene (translate-grid 3 5.5 (hatched-rect3p-x (vec2 0.0 0.0) (vec2 2.0 2.0) 1 0.2)) (translate-grid 5 3.0 (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.5 1.5) 2 0.05)) (translate-grid 2 7.0 (hatched-rect3p-y (vec2 0.0 0.0) (vec2 3.5 3.5) 3 0.5)) (plot-pseudo3d "images/hatching_test/repetition3.png"))
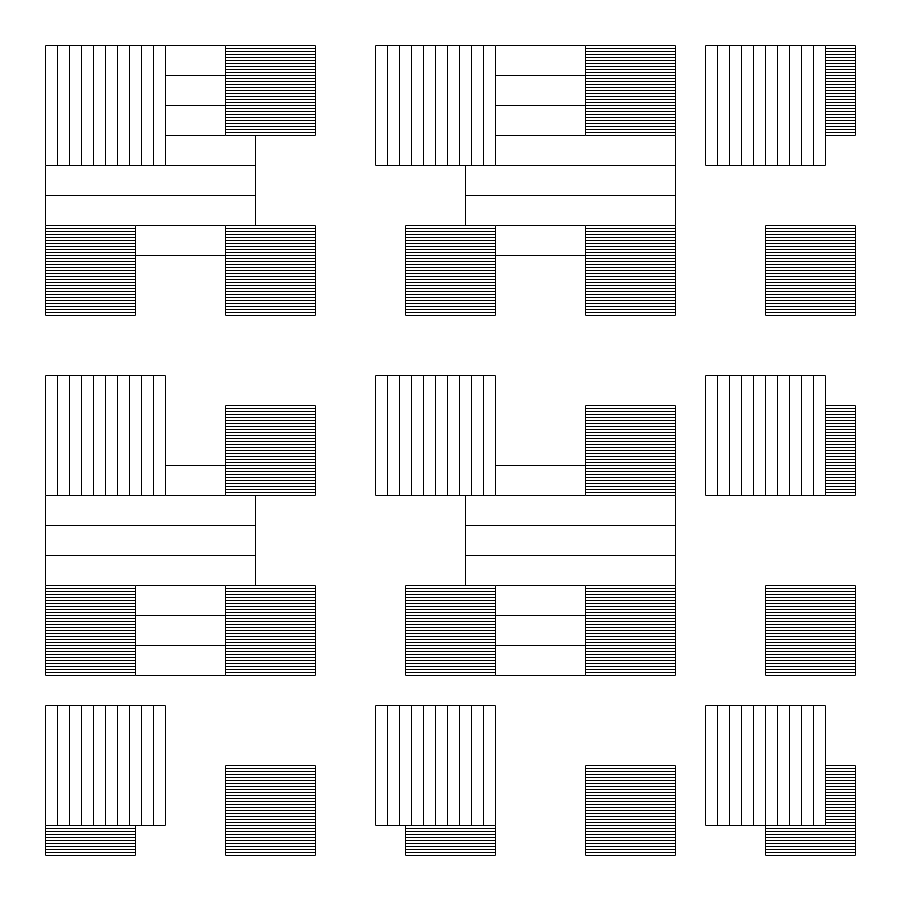
Grids of Grids
(with-scene (translate-grid 5 10.0 (translate-grid 5 3.0 (hatched-rect3p-y (vec2 0.0 0.0) (vec2 1.5 1.5) (rand% 1 1000) 0.5))) (plot-pseudo3d "images/hatching_test/grids2.png"))
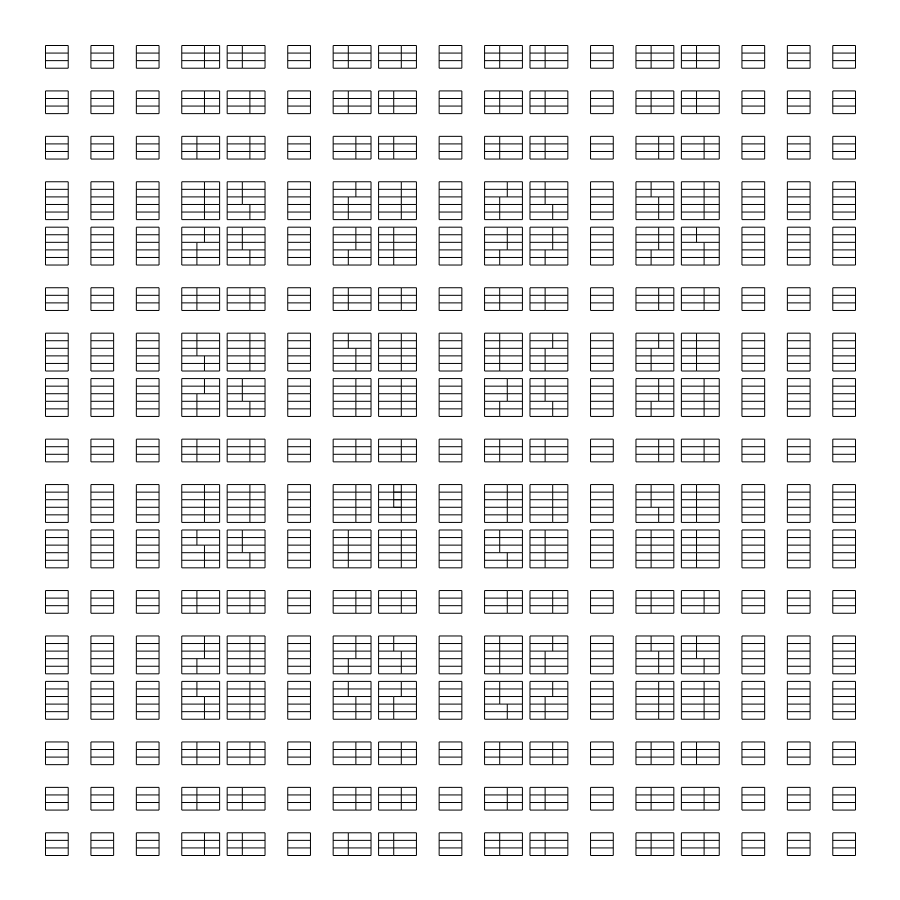
(with-scene (translate-grid 5 15.0 (translate-grid 4 2.5 (rect3p (vec2 0.0) (vec2 3.0) 1))) (translate-grid 3 30.0 (translate-grid 6 3.0 (rect3p (vec2 0.0) (vec2 2.0) 2))) (translate-grid 3 26.0 (translate-grid 5 3.5 (rect3p (vec2 0.0) (vec2 3.0) 0))) (plot-pseudo3d "images/hatching_test/grids3.png"))
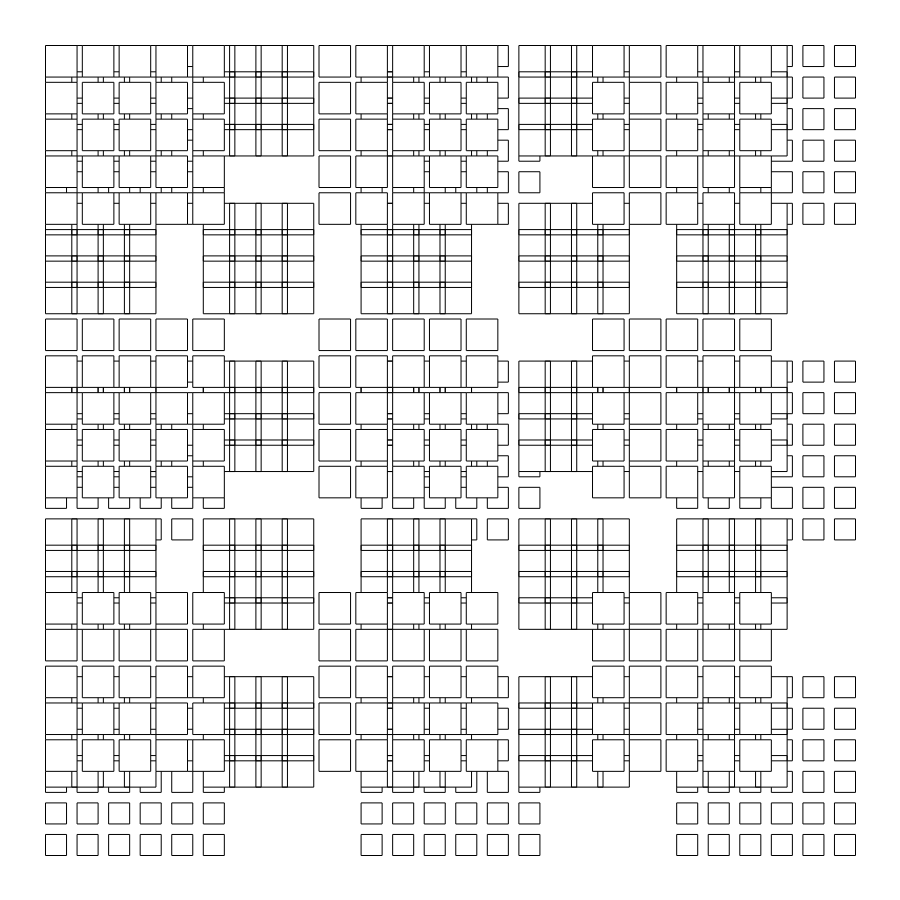